Embarking on the journey of asynchronous programming reveals its critical importance, particularly when navigating the realms of time-intensive operations that are cumbersome and inefficient in a sequential processing landscape. This article serves as the starting point of a potential series, offering a concise introduction to the world of Kotlin coroutines. With these powerful tools, developers are empowered to craft applications that are not only smoother and more responsive but also markedly enhance scalability and user experience. Stay tuned as we explore the foundational aspects of Kotlin coroutines, setting the stage for a deeper dive into more advanced topics in future posts.
What are Coroutines?
A coroutine is a concept akin to a thread but with key differences. It’s a block of code that operates independently of the main execution thread, enabling concurrent operations without blocking the main thread. Typical use cases include data processing, database access, or external API calls.
Coroutines simplify asynchronous programming by enabling code to be written in a sequential style, avoiding the complexity of callbacks, which are prevalent in other asynchronous paradigms (a notable example being JavaScript’s callback hell). This approach enhances code readability and maintainability.
In summary, some of the key features of coroutines are:
- Efficiency: Coroutines are more lightweight than traditional threads. They can be launched in large numbers, running concurrently without burdening the system.
- Non-blocking: The whole idea is to execute code without the need to wait for its completion. Unlike synchronous operations, coroutines allow the main thread to continue its normal execution while the coroutine operations are carried out independently.
- Control over concurrency: Kotlin provides various functions and operators for effectively managing concurrency, such as
launch
,async
,await
,withContext
, and others.
Coroutines vs. Threads: A Comparative Analysis
Both Coroutines and Threads are capable of executing asynchronous tasks, but they are fundamentally different.
Unlike threads, which are execution units managed by the operating system, coroutines are controlled by the Kotlin language runtime, akin to Golang’s goroutines. They are designed for cooperative multitasking, allowing for suspension and resumption of execution, contributing to efficient resource management.
The primary distinction lies in their resource usage. Threads are more resource-intensive, each with its own call stack, and require the operating system to manage their context switching. Coroutines, conversely, are lightweight and operate within threads. Their context switching is managed by the Kotlin runtime, enhancing efficiency. This allows for handling thousands of parallel tasks without system overload, a feat challenging to achieve with threads alone. For more details, refer to this benchmark study.
Hands-on
For our test, we will use a classic algorithm: Fibonacci
The focus here is not the Fibonacci algorithm itself, but keep in mind that it’s a recursive summation algorithm, where each subsequent result depends on the sum of the previous ones. If you’re not familiar with it, check out this link for an explanation. The code below demonstrates a simple implementation of the Fibonacci algorithm. It’s worth noting that there are other ways to implement this algorithm using tail recursion or even matrices, but that’s not very important for our test purpose.
fun fibonacci(num: Int): BigInteger { var a = BigInteger("0") var b = BigInteger("1") var tmp: BigInteger for (i in 2..num) { tmp = a + b a = b b = tmp } return b }
To test the power of running it in parallel we will compare both approaches, parallel and sequential. The idea is to run both methods increasing the length of calculated Fibonnaci value and comparing the time results between them.
fun sequential(length: Int) { for (i in 1..length) { fibonacci(i) } } fun parallel(length: Int) { runBlocking { (1..length).map{ async(Dispatchers.Default) { return@async fibonacci(it) } }.awaitAll() } } fun main(args: Array<String>) { val interactions = args[0].toInt() println("length,sequentialTime,parallelTime") for(length in 1..interactions) { val sequentialStart = System.currentTimeMillis() sequential(length) val sequentialEnd = System.currentTimeMillis() val parallelStart = System.currentTimeMillis() parallel(length) val parallelEnd = System.currentTimeMillis() println("$length,${sequentialEnd - sequentialStart},${parallelEnd - parallelStart}") } }
At the parallel
function we have runBlocking
, async
and Dispatchers.Default
expressions.
runBlocking
: This is a coroutine builder that blocks the current thread until its coroutine and all its children are completed. It’s primarily used for testing or converting regular functions into coroutines. In the context of this code, it ensures that the parallel processing of the Fibonacci sequence is fully completed before moving on.async
: This coroutine builder is used for starting a coroutine that computes a value asynchronously. It returns aDeferred
object, which represents a promise to provide the result later. You can use this to retrieve the result or manage the coroutine’s lifecycle, including cancellation. In our code, it’s used to execute Fibonacci calculations in parallel.Dispatchers.Default
: This is a type ofCoroutineDispatcher
that defines the thread pool where the coroutines will be executed.Dispatchers.Default
is optimized for CPU-intensive work, such as our Fibonacci calculations, by using a shared background pool of threads. This dispatcher enables efficient parallel execution of coroutines. Other dispatchers, likeDispatchers.IO
, are optimized for I/O operations (like DB or filesystem access) and should be chosen based on the nature of the task.
When running the code with increasing values of Fibonacci, we can observe that sequential execution grows more rapidly than running with coroutines. In the end, the sequential calculation took around 350ms while the coroutine-based one took around 50ms. There are some time spikes in both cases, likely due to some other process competing for resources with my script.
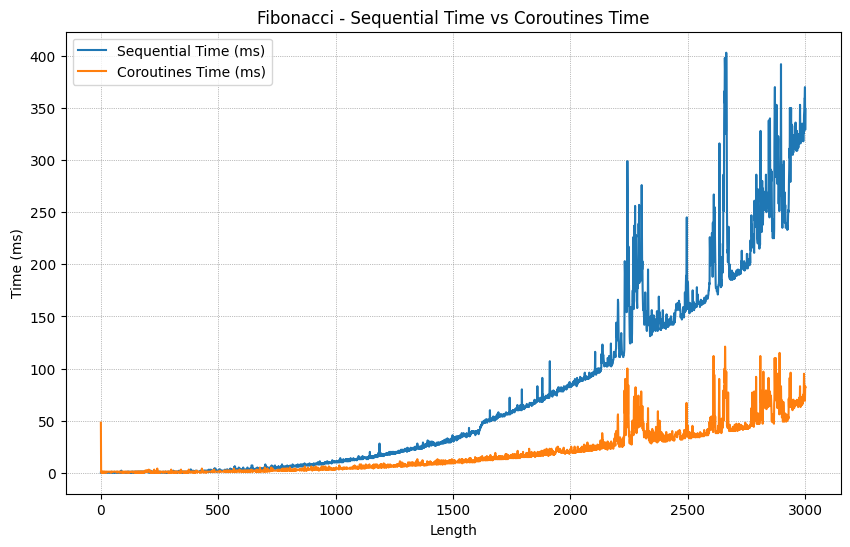
Conclusion
In conclusion, Kotlin Coroutines represents a significant advancement in the realm of app development, offering a more streamlined and powerful approach to asynchronous programming. They not only enhance application performance and scalability, making applications quicker and more responsive but also conserve resources.
This article serves as a brief introduction to the vast and intricate world of Kotlin Coroutines. There is much more to explore beyond the basics covered here, including advanced topics like error handling, the use of channels for communication between coroutines, and strategies for managing shared mutable states. These areas open up even more possibilities, allowing developers to tackle complex programming challenges with greater ease and efficiency.
As we continue to delve into the evolving landscape of technology, understanding and incorporating Kotlin Coroutines is an essential step for developers striving to remain at the cutting edge of efficient and innovative app development.
Links
https://kotlinlang.org/docs/coroutines-overview.html
https://www.techyourchance.com/kotlin-coroutines-vs-threads-memory-benchmark
Be First to Comment